PHP is a popular server-side scripting language used for web development. It is known for its simplicity and flexibility, which makes it a great choice for developing dynamic websites and web applications.
However, like any programming language, PHP has its own terminology that developers must understand to use it effectively. In this blog post, we will provide a comprehensive glossary of terms that every PHP developer should know to master the language.
PHP – PHP (Hypertext Preprocessor) is an open-source, server-side scripting language used for web development. It is widely used to create dynamic web pages.
Server-side scripting – Server-side scripting is a technique in which scripts run on the server, as opposed to the client-side, to generate dynamic web content.
Variable – A variable is a container for storing data in a PHP program. Variables are named memory locations used to hold values that can be changed throughout the execution of a program.
Data types – Data types refer to the different types of data that can be stored in a variable, such as integers, floating-point numbers, strings, arrays, and objects.
Operators – Operators are symbols or keywords used in PHP to perform mathematical, logical, or string operations.
Control structures – Control structures are statements in PHP that control the flow of a program. They include if/else statements, switch statements, loops, and function calls.
Function – A function is a block of code in PHP that performs a specific task. Functions are reusable, making them a fundamental building block of PHP programming.
Arrays – Arrays are a collection of data items in PHP that are stored under a single variable name. They can be indexed numerically or using strings.
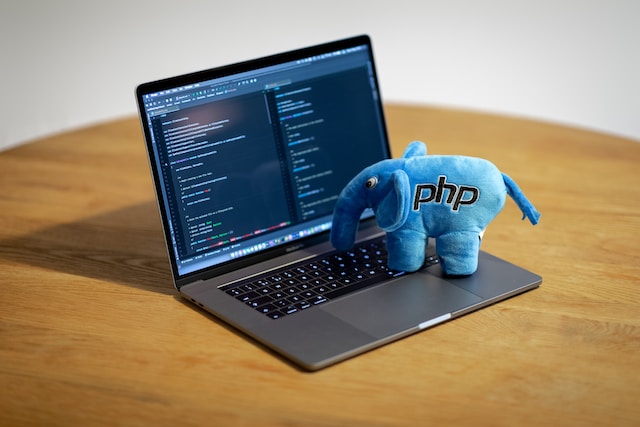
Object-oriented programming (OOP) – OOP is a programming paradigm that uses objects to represent and manipulate data. It provides encapsulation, inheritance, and polymorphism as key concepts for organizing code.
Class – A class is a blueprint for creating objects in PHP. It defines the properties and methods that an object of that class can have.
Inheritance – Inheritance is a concept in OOP that allows a subclass to inherit properties and methods from its parent class. It promotes code reuse and simplifies program design.
Polymorphism – Polymorphism is a concept in OOP that allows objects to take on multiple forms. It allows methods to be overridden in a subclass to provide different functionality.
Interface – An interface is a template for implementing a set of methods that a class must define. It defines a contract for the behavior of an object, without specifying its implementation.
Namespace – A namespace is a way to group related classes, functions, and constants in PHP. It prevents naming collisions and makes code more organized and modular.
Exception – An exception is a run-time error that occurs during the execution of a PHP program. It can be caught and handled by the program to prevent the program from crashing.
Constant – A constant is a value that cannot be changed during the execution of a PHP program. Constants are defined using the “define()” function and are often used to store configuration settings and other global values.
Session – A session is a way to store data between page requests in a PHP application. It allows developers to keep track of user data and state, even when the user navigates away from the current page.
Cookie – A cookie is a small piece of data stored on the user’s computer by a website. Cookies are often used to remember user preferences or login information.
File handling – File handling is the process of reading from and writing to files in a PHP program. PHP provides a variety of functions for working with files, including “fopen()”, “fwrite()”, and “fclose()”.
Regular expression – A regular expression is a pattern used to match strings in a PHP program. Regular expressions are often used for tasks such as data validation and parsing.
Database – A database is a structured collection of data that can be accessed, managed, and updated. PHP provides support for a variety of databases, including MySQL, PostgreSQL, and MongoDB.
MySQL – MySQL is a popular open-source relational database management system used with PHP. It provides a wide range of features, including support for transactions and advanced security.
PDO – PDO (PHP Data Objects) is a PHP extension that provides a consistent interface for accessing databases. It supports a wide range of databases, including MySQL, PostgreSQL, and SQLite.
MVC – MVC (Model-View-Controller) is a software architecture pattern used in PHP web development. It separates an application into three components: the model (data), the view (presentation), and the controller (logic).
Framework – A framework is a collection of libraries and tools used to build web applications in PHP. Popular PHP frameworks include Laravel, Symfony, and CodeIgniter.
Templating engine – A templating engine is a tool used to separate the presentation layer from the logic layer in a PHP application. It allows developers to create reusable templates for displaying data, improving code organization and reducing code duplication.
RESTful API – A RESTful API (Representational State Transfer Application Programming Interface) is a web-based API that uses HTTP requests to perform CRUD (Create, Read, Update, Delete) operations on data. It is a popular approach for building web services and mobile applications.
Composer – Composer is a dependency manager for PHP that allows developers to easily manage dependencies in their projects. It provides a simple way to install, update, and manage third-party libraries and packages.
Autoloading – Autoloading is a feature in PHP that automatically loads classes and files when they are needed, without requiring the developer to manually include them.
Dependency Injection – Dependency Injection is a design pattern in which dependencies are injected into an object instead of being created inside the object. It promotes loose coupling and improves testability and maintainability of code.
Testing – Testing is the process of verifying that a PHP application behaves as expected under different conditions. PHP provides a range of testing tools and frameworks, including PHPUnit and Behat.
Debugging – Debugging is the process of finding and fixing errors in a PHP application. PHP provides a range of debugging tools, including Xdebug and Zend Debugger.
Security – Security is a critical concern in PHP web development. Best practices include input validation, secure database queries, and protecting against common web attacks such as SQL injection and cross-site scripting (XSS).
Internationalization – Internationalization (i18n) is the process of adapting a PHP application to support multiple languages and cultural conventions. PHP provides a range of tools and libraries for i18n, including gettext and the Internationalization extension (intl).
Localization – Localization (l10n) is the process of adapting a PHP application to the specific language and cultural conventions of a particular region or locale. It involves translating text, formatting numbers and dates, and adapting other aspects of the application’s user interface.
Caching – Caching is the process of storing frequently used data in memory or on disk to improve performance. PHP provides a range of caching tools and libraries, including APCu and Memcached.
Content Management Systems – Content Management Systems (CMS) are web applications that allow users to create, manage, and publish content on the web. Popular PHP CMSs include WordPress, Drupal, and Joomla!.
E-commerce – E-commerce refers to the buying and selling of goods and services over the internet. PHP provides a range of tools and libraries for building e-commerce applications, including payment processing and shopping cart functionality.
Web Services – Web services are software systems that allow communication between different applications over the internet. PHP provides a range of tools and libraries for building web services, including SOAP and RESTful APIs.
Scalability – Scalability is the ability of a PHP application to handle increased traffic and workload. PHP provides a range of tools and techniques for improving application scalability, including load balancing, database sharding, and caching.
Asynchronous programming – Asynchronous programming is a programming model in which tasks are executed concurrently, allowing for improved performance and responsiveness. PHP provides support for asynchronous programming through extensions such as ReactPHP and Amp.
Server-side rendering – Server-side rendering is the process of generating HTML on the server and sending it to the client, as opposed to generating HTML on the client side using JavaScript. PHP provides a range of tools and libraries for server-side rendering, including the Twig templating engine and the Symfony framework.
Microservices – Microservices are a software architecture pattern in which an application is broken down into smaller, independent services that communicate with each other over a network. PHP provides a range of tools and libraries for building microservices, including the Slim framework and the Lumen micro-framework.
Continuous integration/continuous delivery – Continuous integration/continuous delivery (CI/CD) is a software development process in which code changes are automatically tested and deployed to production. PHP provides a range of tools and libraries for CI/CD, including Jenkins, Travis CI, and GitLab CI.
Virtualization – Virtualization is the process of creating a virtual version of a resource, such as a server or operating system. PHP provides a range of tools and libraries for virtualization, including Docker and Vagrant.
DevOps – DevOps is a software development methodology that emphasizes collaboration between development and operations teams. PHP provides a range of tools and libraries for DevOps, including Ansible and Chef.
Cloud computing – Cloud computing refers to the delivery of computing services over the internet. PHP provides a range of tools and libraries for cloud computing, including AWS SDK for PHP and Google Cloud Platform.
Serverless computing – Serverless computing is a cloud computing model in which the cloud provider manages the infrastructure and automatically scales resources based on demand. PHP provides support for serverless computing through extensions such as AWS Lambda and Google Cloud Functions.
Artificial intelligence/machine learning – Artificial intelligence/machine learning refers to the use of algorithms and statistical models to analyze data and make predictions or decisions. PHP provides a range of tools and libraries for AI/ML, including the PHP-ML machine learning library and the TensorFlow PHP extension.
Blockchain – Blockchain is a distributed ledger technology that provides a secure, transparent, and tamper-proof way of storing and sharing data. PHP provides support for blockchain development through libraries such as PHP-Ethereum and PHP-bitcoinrpc.
Object-Relational Mapping (ORM) – ORM is a technique used to map relational database concepts to object-oriented programming concepts. This allows developers to interact with databases using objects, rather than writing raw SQL queries. PHP provides a range of ORM frameworks, including Doctrine and Propel.
Websockets – Websockets is a technology that allows for real-time, bi-directional communication between a client and a server. PHP provides support for Websockets through libraries such as Ratchet and Socket.IO.
Reactive Programming – Reactive programming is a programming paradigm that emphasizes asynchronous and event-driven programming. Reactive programming is useful for building responsive, scalable applications that can handle large numbers of requests. PHP provides support for reactive programming through libraries such as ReactPHP and RxPHP.
Static Site Generators – Static site generators are tools that allow developers to create websites that consist of static files. This approach is faster, more secure, and easier to deploy than traditional dynamic websites. PHP provides support for static site generators through tools such as Sculpin and Jekyll-PHP.
Functional Programming – Functional programming is a programming paradigm that emphasizes the use of pure functions and immutable data structures. Functional programming is useful for building applications that are easier to reason about, test, and debug. PHP provides support for functional programming through libraries such as RamdaPHP and Functional PHP.
Machine-to-Machine (M2M) Communication – M2M communication refers to the communication between devices over a network, without human intervention. PHP provides support for M2M communication through libraries such as MQTT-PHP and CoAP-PHP.
Command-Line Interfaces (CLI) – Command-line interfaces are tools that allow developers to interact with an application through a command-line interface, rather than a graphical user interface. PHP provides support for building CLI applications through the Symfony Console component and the Laravel Artisan CLI.
Natural Language Processing (NLP) – NLP is a branch of artificial intelligence that deals with the interaction between computers and human language. PHP provides support for NLP through libraries such as PHP-ML and Natural Language Toolkit (NLTK).
Big Data – Big Data refers to the processing and analysis of large, complex data sets. PHP provides support for Big Data through libraries such as Apache Spark-PHP and Hadoop-PHP.
Chatbots – Chatbots are software programs that interact with users through a messaging interface. PHP provides support for building chatbots through libraries such as BotMan and Wit-PHP.